README.md (9062B)
1 # roast for secp256kfun [frost](https://github.com/LLFourn/secp256kfun/blob/master/schnorr_fun/src/frost.rs) 2 ## unfishished and not ready for use 3 4 5 Click [here to get an idea for how this roast wrapper can be used](https://github.com/nickfarrow/roast/blob/master/roastlib/src/main.rs) 6 7 `endpoints/` is not currently being developed, just the core `roastlib/`. 8 9 [roast paper](https://eprint.iacr.org/2022/550.pdf) 10 11 ## todo 12 Later will be made agnostic to the threshold signature scheme that is used / frost implementation that is used. 13 14 Finish roastlib and test without specifying any io methods from client to server 15 16 Create communication endpoints for both ROAST server (coordinator) and signer clients using new layout, then use them to communicate a test session. 17 * Current plan is to communicate via http requests and rocket with some mutable state for client and server. 18 19 ## excerpt 20 ``` 21 let frost = secp_frost::Frost::<Sha256, Deterministic<Sha256>>::default(); 22 let (secret_shares, frost_keys) = frost::frost_keygen(2, 3); 23 24 let message = Message::plain("test", b"test"); 25 let roast = coordinator::Coordinator::new(frost.clone(), frost_keys[0].clone(), message); 26 27 // Create each signer session and create an initial nonce 28 let (mut signer1, nonce1) = signer::RoastSigner::new( 29 frost.clone(), 30 frost_keys[0].clone(), 31 0, 32 secret_shares[0].clone(), 33 [].as_slice(), 34 message, 35 ); 36 let (mut signer2, nonce2) = signer::RoastSigner::new( 37 frost, 38 frost_keys[1].clone(), 39 1, 40 secret_shares[1].clone(), 41 [1].as_slice(), 42 message, 43 ); 44 45 // Begin with each signer sending a nonce to ROAST 46 let (combined_signature, nonce_set) = roast.process(0, None, nonce1); 47 assert!(nonce_set.is_none()); 48 assert!(combined_signature.is_none()); 49 50 let (_combined_signature, nonce_set) = roast.process(1, None, nonce2); 51 assert!(nonce_set.is_some()); 52 53 // Once ROAST receives the threshold number of nonces, it responds with a nonce set 54 let sign_session_nonces = nonce_set.expect("roast responded with nonces"); 55 56 // The signer signs using this the nonces for this sign session, 57 // and responds to ROAST with a signature share. 58 let (sig_share2, nonce2) = signer2.sign(sign_session_nonces.clone()); 59 let (combined_signature, nonce_set) = roast.process(1, Some(sig_share2), nonce2); 60 dbg!(&combined_signature.is_some(), &nonce_set.is_some()); 61 assert!(combined_signature.is_none()); 62 63 // ROAST also sends the nonce set to the other signer, who also signs 64 let (sig_share1, nonce1) = signer1.sign(sign_session_nonces); 65 66 let (combined_signature, nonce_set) = roast.process(0, Some(sig_share1), nonce1); 67 dbg!(&combined_signature.is_some(), &nonce_set.is_some()); 68 assert!(combined_signature.is_some()); 69 70 // Once the threshold number of signature shares have been received, 71 // ROAST combines the signature shares into the aggregate signature 72 dbg!(combined_signature); 73 ``` 74 75 ## ROAST Paper Notes 76 [roast paper](https://eprint.iacr.org/2022/550.pdf) 77 78 Roast is a simple wrapper that turns a given threshold signature scheme into a scheme with a robust and asynchronous signing protocol, as long as the underlying signing protocol is semi-interactive (i.e. has one preprocessing round and one actual signing round), proviceds identifiable aborts, and is unforgable under concurrent signing sessions. 79 80 81 Robustness is the guarentee that t honest signers are able to obtain a valid signature even in the presence of other malicious signers who try to disrupt the protocol. 82 83 84 FROST provides identifiable aborts (IA): if signing session fails, then honest signers can identify at least one malicious signer responsible for the failure. 85 86 We cant run every combination of signers n choose t, too computationally expensive -> ROAST tackles this problem. 87 >an algorithmic approach to choosing signer sets based on past behaviour? 88 89 90 91 92 ### Security of Threshold Signatures 93 94 Identifiable aborts: 95 - Ensures that ShareVal reliably identifies disruptive signers who send wrong shares. The IA-CMA (identifiable abort, chosen message attack) game: A controls all but one signer and can ask the remaining honest signer to take part in arbitrary number of concurrent sign sessions. Wins if the malicious signers all submit presignature or signature shares that somehow pass validation but lead to an output of an invalid signature (break of accountability). Or A wins if the honest signer outputs a presignatures and signature shares that will not pass validation. 96 97 98 Unforgability: a threshold signature scheme is existentially unforgable under CMA and concurrent sessions if no adversary A which controls t-1 signers during keygen and signing and can ask the remaining n-t+1 honest signers to take part in arbitrarily many concurrent signing sessions on messages of its choice, 99 100 -> ie.e for every honest signer, A has oracles simulating PreRound(PK) and SignRound(sk_i, PK, State_i_sid) on an already preprocessed but unfinished session sid of its choice.can 101 102 can produce a valid signature on a message that was never used in a signing session and A never asked in any query round. 103 104 105 #### Nonce aggregation 106 A semi-interactive threshold signature scheme is aggregatable if |p| and |sigma| are constant in parameters n and t, for p <- PreAgg(PK, {t_i}_i_in_T), sigma <- SignAgg(PK, p, {sigma_i}_i_in_T) and all inputs PK and m. 107 108 The aggregation of these elements is important for practical purposes as it reduces the size of the final signature as well as the amount of data that needs to be breadcast during signing. 109 110 In each of the signing rounds, a coordinator node will be one of the ssigners and can collect the contributions from all signers, aggregate them, and broadcast only the aggregate output back to signers. 111 112 > Doesn't seem super important 113 114 FROST3 -> PreAgg (nonce agg) -> Aggregate two presignature products D=prod(d_i), and E=prod(e_i) for i in T. Whereas FROST2 the aggregated presignature is not really aggregated, just the set {(D_i, E_i) for i in T}. The SignRound algorightm takes care of computing the products, as before. Other FROST versions include 2-BTZ and 2-CKM. 115 116 ### FROSTLAND 117 A majority of t of 15 council members is needed to sign a bill for it to pass. 118 119 Each counci member has its own twatermark and a bill is only vaild if it carries the watermarks of all signers (and no others). 120 121 Find a majority of council members, use thier watermarks to create the paper, then collect their signatures. However if one of them fail to sign at the final step, then the process talls. It is not possible to ask anyone else since the watermark on the page corresponds to the disruptive signer. So we must start the signing process from scratch. 122 123 From time to time, members try to disrupt the signing process in an attempt to prevent other members from passing the bill and refuse to sign even though they indicated support. 124 125 The solution process is the following procedure: 126 In the beginning, all the council members that signal support for the bill are asked to gather. The secretary maintains a slist of all these members and whenever there are at least 9 members on the list, they call a group of 9 members to their office and strikes out their names on the list. 127 128 He then obtains paper with the watermarks of those 9 members, writes a copy of the bill and askes them each to sign. Whenever a council member has completed signing the copy they leave the office and the secretary adds their name back to the list. 129 130 If at least 9 council members behave honestly then they will eventually sign their assigned copy and be readded to the list. WIll this procedure we know that these 9 members will be on the list at some point in the future and so the signing procedure will not get stuck. Since members are assigned a new copy each time, a member can at most hold up the singing of at most one copy at a time. The naximum n-t 1= 15-9 = 6 disruptive council members can hold up 6 copies at most. 131 132 ### Robust Asychronous Threshold Signatures 133 134 The coordinators task is to maintain a set of responsive signers who have responded to all previous signing requests. As soon as R contains t, C initiates a new signing session. 135 136 Along with each signature share, each signer is also required to provide a fresh presignature share (nonce) in preparation for a poosible next signing session. "A pipeline of signing sessions" 137 138 139 ### Eliminating the Trusted Coordinator 140 A simple method to eliminate the need for semi-strusted coordinator is to let the signers run enough instances of the coordinator process: the n signers choose among themselves any set n-t+1 coordinators and start s-t+1 concurrent runs of roast. Note that one of these sessions will have t honest signers. 141 142 The concurrent runs of ROAST do not need to be started simultaneously - e.g. honest signers can resend their reply in the run with coorinator_2 only after d seconds and only if they have not obtained a valid signature from any other run (is that a concern? Yes excessive computation and communication.) 143 144 145 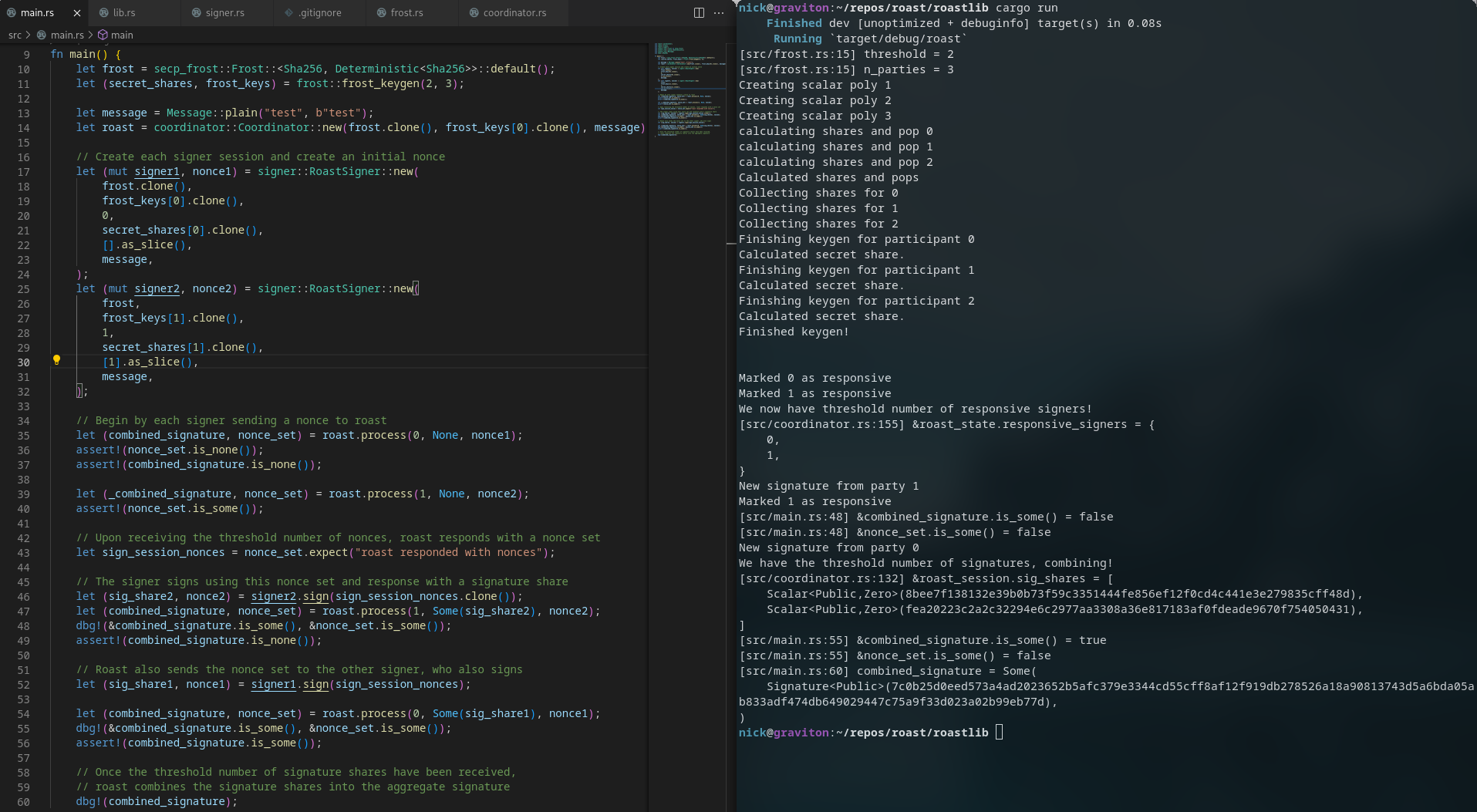